フラッシュサイトの専売特許だったNowローディング(プリローダとも)ですが、jQueryやHTML5の登場で、最近では、非フラッシュサイトでも見かけるようになりました。この記事では、jQueryを使ってプレローダーを表示する方法を考えて見ます。
デモ
今回作るプリローダーのデモです。一度読み込むとキャッシュされてしまうので、もう一度みたい方は、ブラウザをキャッシュを消して見てください。
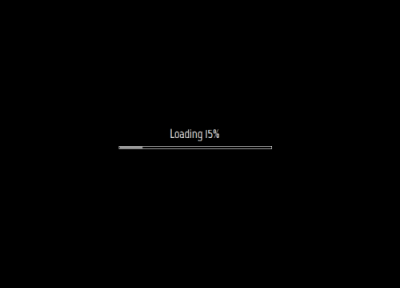
考え方とコード
イメージファイルが重いサイトの場合であれば、イメージをプレローディングしながら、読み込んだイメージ数に応じた%を表示することでプリロード します。すべて読み込んだらメインコンテンツを表示します。
このサンプルコードでは、画像のロードイベントを拾って読み込んだイメージをカウントし、%とプログレスバーの表示を変えています。
<!DOCTYPE html> <html> <head> <script src="js/jquery.js"></script> <script> $(function() { Array.prototype.remove = function(element) { for (var i = 0; i < this.length; i++) if (this[i] == element) this.splice(i,1); }; function preload(images, progress) { var total = images.length; $(images).each(function(){ var src = this; $('<img/>') .attr('src', src) .load(function() { images.remove(src); progress(total, total - images.length); }); }); } preload([ 'img/DSC00001.JPG', 'img/DSC00018.JPG', 'img/DSC00222.JPG', 'img/DSC00845.JPG', 'img/DSC00886.JPG', 'img/DSC00939.JPG', 'img/DSC02908.JPG' ], function(total, loaded){ if (loaded >= total) { // すべて読み込んだ $('#loader').fadeOut('slow', function() { $('<img />') .attr('src', '/demo/20111116/img/DSC00018.JPG') .appendTo('#content'); $('#content').fadeIn('slow'); }); } else { // 読み込み途中 var percent = Math.ceil(100 * loaded / total); $('#load-text').html(percent + '%'); $('#bar span').css('width', percent + '%'); } }); }); </script> </head> <body> <div id="loader"> Loading <span id="load-text">0%</span> <div id="bar"><span></span></div> </div> <div id="content" style="display: none;"> <p>Here is content</p> </div> </body> </html>
滑らかにする
先ほどのデモを見るとわかりますが、画像の枚数が少ないとカウントアップが雑になったり、一度キャッシュができてしまうと、ローディングが見えなかったりします。
そこで、スムーズに1%ずつカウントアップするバージョンを考えてみました。
表示しているパーセント が、現時点での読み込みパーセントを1%ずつ追う仕組みになっています。
<!DOCTYPE html> <html> <head> <script src="js/jquery.js"></script> <script> $(function() { Array.prototype.remove = function(element) { for (var i = 0; i < this.length; i++) if (this[i] == element) this.splice(i,1); }; function preload(images, progress) { var total = images.length; $(images).each(function(){ var src = this; $('<img/>') .attr('src', src) .load(function() { images.remove(src); progress(total, total - images.length); }); }); } var now_percent = 0; var displaying_percent= 0; preload([ 'img/DSC00001.JPG', 'img/DSC00018.JPG', 'img/DSC00222.JPG', 'img/DSC00845.JPG', 'img/DSC00886.JPG', 'img/DSC00939.JPG', 'img/DSC02908.JPG' ], function(total, loaded){ now_percent = Math.ceil(100 * loaded / total); }); var timer = window.setInterval(function() { if (displaying_percent >= 100) { window.clearInterval(timer); $('#loader').fadeOut('slow', function() { $('#content').fadeIn('slow'); }); } else { if (displaying_percent < now_percent) { displaying_percent++; $('#load-text').html(displaying_percent + '%'); $('#bar span').css('width', displaying_percent + '%'); } } }, 20); // この数字を変えるとスピードを調整できる }); </script> </head> <body> <div id="loader"> Loading <span id="load-text">0%</span> <div id="bar"><span></span></div> </div> <div id="content" style="display: none;"> <p>Here is content</p> </div> </body> </html>
53行目の数字は、%表示の更新間隔(ミリ秒)です。小さくするとスピードがあがり、大きくするとゆっくりになります。使うときは調整してみてください。
ダウンロード
デモファイルはこちらからダウンロードできます。jQueryのプラグインとして実装もできそうですので、興味がある人はやってみてください。
コメント